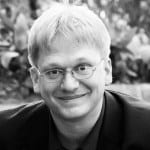
D Programming: Best Tutorials to Get Started
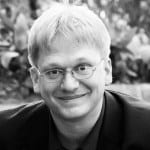
Disclosure: Your support helps keep the site running! We earn a referral fee for some of the services we recommend on this page. Learn more
What comes after C? Well, actually C++, C#, and Objective-C. But then D.
D is a relatively new programming language. It was designed as an attempt to keep all the good things from C, and add in the benefits from the C-derived languages (mostly C++).
However, whereas C++ maintained backward compatibility with C, D does not attempt to do so. This allows D to leave behind what the designers call C’s “weaknesses.”
History of D
Walter Bright began working on D in 1999. Bright is the owner of Digital Mars, a software company that makes compilers. The first public release of D was in 2001, but the v1.0 release didn’t come until 2007.
By the time of the v1.0 release, the language already had a dedicated user base — and an opinionated one at that. The community was widely unhappy with D’s standard library (called “Phobos”) and developed a replacement for it (“Tango”), which was released in 2007 as well.
2007 also saw the introduction of D2, a backwards incompatible major version (which is now the canonical “D”). The Phobos library was included as part of the D2 release, and it took the Tango community until 2012 to complete a port of Tango to D2.
D2 stabilized around 2010, and in 2011 development of the language moved to GitHub.
These events, along with release of the definitive book on D, by one of its developers, spurred rapid growth in the developer community. Since then, the language has gotten more and more attention and serious use.
About the Language
D is based on C and C++. It is designed to look and feel like those languages, making it easy for C/C++ developers to transition to D. Syntax that is valid in C or C++ and D should do the exact same thing.
General Principles
D is intended to be as easy to use as possible, particularly for C/C++ and Java developers.
It provides high-level constructs and abstractions that do not exist in C or C++, but still allows for “bare metal” access — the ability to literally flip bits on hardware, and do other extremely low level programming.
This is intended to combine the benefits of higher-order languages like Python with the raw power of C. Since it is a compiled language, it also has the runtime speed of C and C++.
D is designed to support several major programming paradigms: object oriented, functional, imperative, concurrent, and metaprogramming.
The design of D specifically leaves out a number of C and C++ language features, including:
- multiple inheritance
- namespaces
- forward declarations
- includes
- trigraphs and digraphs
- bit fields.
Major Features
Noteworthy features of D include:
- Classical Object Orientation, with single-inheritance and interfaces to provide most of the benefits of multiple inheritance.
- Operator Overloading, the ability to design classes that implement their own methods for operators (
+
,-
,*
,/
). This allows you to, for example, define what it means to add two non-numerical things together.
- Operator Overloading, the ability to design classes that implement their own methods for operators (
- Functional Programming:
- lambdas
- closures
- immutable data structures
- pure (side-effect free, stateless) functions.
- Advanced function handling, including:
- nested functions
- function literals
- function overloading
- virtual functions.
- Importable modules.
- Templated programming.
- In-source documentation.
- Improved arrays:
- arrays are First-class objects
- array dimensions are available from the array
- arrays are resizable
- arrays can be bounds checked
- several different array types available: pointers, static arrays, dynamic arrays, and associative arrays.
- Improved string handling.
- Ranges.
- Automated garbage collection, with the ability to explicitly control memory allocation and deallocation if needed.
- Contracts.
- Built-in unit tests.
- Try-Catch-Finally exception handling
D Resources
Online
Official D Links
- The Official D Website
- Digital Mars: the company behind D, and the maker of the reference compiler
- The D Forum: discussion board.
- Defunct
- D1 Website: language reference for v1 of the language. This is helpful if you are dealing with legacy D code. Also it can provide insight into the thinking behind the language, as there is a lot of material documenting its development.
Tutorials / Learning
- D: A Newbie-Oriented Tutorial: geared for people learning D as a first language, or with limited programming ability
- A Beginner’s Guide to D: a Wikibook, geared for people who have some programming ability but not with C or C++
- D Transition Guide: a short tutorial on D, for experienced C/C++ developers
- Development With D: a guide to finding D programming resources
- Pragmatic D Tutorial
- D Programming Basics
- Beginner Tutorial
- DFL Tutorial
- D Templates Tutorial
- Diving into the D Programming Language
- D Bare Bones
- D (The Programming Language)
- D Tutorial
- D Programming Language: Getting started (video)
- A Real D In Programming (video).
Tools
Books
- The D Programming Language
- Learning D
- Learn to Tango with D
- D Cookbook
- Programming in D: Tutorial and Reference: for those learning to program, with D as a first language; Also available for free online
- D Web Development.
Should I learn D?
D is a powerful language, designed for people who build software systems and code every day.
If you are just learning to code, D is probably not for you. (The D language documentation specifically suggests Python or JavaScript for first-time programmers.)
If you already use C, C++, or Java, you are probably a good candidate for D: it will make sense to you, you’ll appreciate its improvements, and your work will benefit from its increased power and ease of use.
Further Reading and Resources
We have more guides, tutorials, and infographics related to coding and development:
- C++ Developer Resources: if you’d rather stick to a more traditional language, this page provides you with all the tools you need.
- Objective-C Guide and Resources: yet another C-like language with object-oriented elements, Objective-C is very important in development for Apple products.
- Swift Introduction and Resources: this is one of the newest C-like languages. The future of programming? Find out here!
What Code Should You Learn?
Confused about what programming language you should learn to code in? Check out our infographic, What Code Should You Learn?
It not only discusses different aspects of the languages, it answers important questions such as, “How much money will I make programming Java for a living?”
Comments