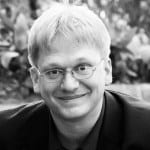
Objective-C Development: Get Started Building Mac and iOS Apps
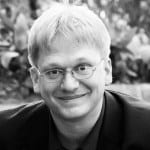
Disclosure: Your support helps keep the site running! We earn a referral fee for some of the services we recommend on this page. Learn more
Objective-C is a high-level programming language based on C, with additional features and syntax from Smalltalk. It is a superset of the C language, which means that any valid C code will run in an Objective-C compiler. This was an intentional decision made by the designers of the language, who wanted to make sure that the language was backwards-compatible with existing C applications and components, since that language was used for the majority of operating system and utilities programming up to that point (the early 1980s).
Short History of Objective-C
Objective-C was invented in the early 1980s as a means to add Object Oriented programming capabilities to C. The inventors, Brad Cox and Tom Love, thought that Smalltalk (an early OO language) could provide the tools needed for truly re-usable code and for creating development environments for systems developers.
Cox began by writing a pre-processor for C that allowed for the inclusion of Smalltalk-like code, which would then be rendered into compilable C. This turned into a fully-Object-Oriented C extension.
Objective-C was eventually fully defined and described in the book Object-Oriented Programming: An Evolutionary Approach.
NeXT licensed Objective-C in 1988 and developed several tools based on it. These tools eventually became (after several intermediate steps) the Cocoa development environment, which is used by several Apple systems:
Mac OSX
iOS
Apple WatchOS
For several years now, apps written for one of these Apple operating systems needed to be written (or compiled to, or run on top of) Objective-C. That situation is changing now as Apple moves its platform to Swift.
In addition to Cocoa, Objective-C is also used in the GNUstep platform, which can run on Linux, Unix, BSD, and Windows environment, and which closely mirrors Cocoa.
About the Objective-C language
Objective-C is one of two major Object Oriented derivations of C, the other being C++. Each language took the underlying language and implemented Object Orientation differently. (More recent versions of C have followed the lead of C++, rather than Objective-C, in their implementation of object orientation. This has led to further divergence between C and Objective-C.)
Messaging
In most Object Oriented Languages, including C++,functions are invoked by calling a method on an object. This method of invocation derives from Simula, the first Object Oriented language.
Objective-C’s syntax for invoking a function comes from Smalltalk, where a message is passed to an object.
This difference might seem trivial, but it has a number of implications. Significantly, the function call can be tied to a specific object at runtime, rather than at compile time, allowing for more flexible implementations.
Dynamic Typing
Objective-C, like its predecessor Smalltalk, can take advantage of dynamic typing. This means that an object can receive messages not specified in the class interface — something that would cause an error or exception in C++ and many other languages.
Because the message is not directly a method call, the object is not required to have a method to run. It can pass the message on to another object, via message forwarding, or it can respond to the message in some other way, or raise an error. This allows easier implementation of several design patterns, including the proxy pattern and the observer pattern.
Categories
Categories are a sort of superclass structure that allows methods to be added to classes at runtime. This allows methods to be added to classed without recompiling those classes, or even having source code access. They can also replace existing class methods.
This feature eases maintenance and expansion of large, complicated code bases.
Online Objective-C Resources
Below are resources to help you learn and use Objective-C. They are subdivided into different categories so you can more easily find the information you are looking for — whether programming basics or advanced education or Objective-C tools.
Objective-C Tutorials and Intro Material
These documents will get you started as an Objective-C programmer — even if you start with no experience with the language. So if you are just starting out, this is where you should begin.
Introduction to Objective-C — An overview of Objective-C from Apple.
Objective-C Introduction — A basic overview of Objective-C and the OpenStep platform, from a developer involved with high-performance mathematics.
Basic Programming Concepts for Cocoa and Cocoa Touch — Introduction to the Cocoa environment.
Introduction to Coding Guidelines for Cocoa — General information on the Cocoa API, which uses Objective-C.
Writing Documentation for Objective-C — The NSHipster guide to Objective-C documentation. (That is, documentation written about code, not about the language.)
The Beginner’s Guide to Objective-C: Language and Variables — A beginner’s Objective-C tutorial from Treehouse.
Try Objective-C — A free, five-part class on Objective-C from Code School.
Learn Objective-C in 24 Days — A massive, 24-part course on Objective-C, from Feifan Zhou. (Also see this complete list of Objective-C tutorials on binpress.)
Learn Objective-C — Another multi-part Objective-C course, from tuts+.
Cocoa Dev Central — An illustrated tutorial on Objective-C.
Ry’s Objective-C Tutorial — a concise quick-reference and a comprehensive introduction for newcomers to the language.
Objective-C Tutorial — A decent (not amazing) tutorial from tutorialspoint.
Objective-C Tools
The following Objective-C tools will make coding easier and more powerful. That’s particularly true with the Objective-C tools available in the Apple Developer Network, which costs $99 per year, but is nonetheless well worth it.
LispWorks for Mac — Includes an API for creating Objective-C classes and call methods — see the LispWorks Objective-C and Cocoa User Guide and Reference Manual for more details.
Objective-C Editor — more an integrated development environment than an editor, it allows you to do all our work in one place.
Apple Developer Network — although not a tool itself, the Apple Developer Network provides access to many great Objective-C tools like the Xcode IDE and the Foundation Framework.
Codebeat — an analysis system to help you improve your code with a focus on web and mobile development.
Reference
The following documents provide code snippets, cheat sheets, and hypertext documents to make Objective-C coding easier.
Objective-C Cheat Sheet — A quick reference cheat sheet for common, high level topics in Objective-C.
NSHipster Fake Book — Over 200 Objective-C licks for iOS and MacOS X developers.
Objective-C Runtime Reference — From Apple, all about OS X Objective-C 2.0 runtime library support functions and data structures.
Objective-C Cheat Sheet and Quick Reference — One page PDF covering common Objective-C issues. Great for hanging on the wall next to your dev machine.
Publishers
Here are a couple of publishers that create content of interest to Objective-C programmers.
Objc.io — objc.io publishes books on advanced techniques and practices for iOS and OS X development.
NSHipster — a journal of the overlooked bits in Objective-C, Swift, and Cocoa.
Books about Objective-C
For those who like to really dive into a subject, here are some of the best books available about Objective-C. They include books for those just starting out, those who wish to increase their knowledge, and those who need reference material.
Introductory Books
Advanced Books
Reference Books
Swift — The Successor to Objective-C
If you are learning Objective-C, it is probably also a good idea to begin learning Swift.
The primary use for Objective-C is apps in Apple’s Cocoa platform, on their three operating systems (OS X, iOS, WatchOS). Apple has announced that these platforms will now switch to their new programming language, Swift.
Here are a few Swift resources:
The Swift Programming Language — The introductory guide to Swift, from Apple. Also see the iBooks book of the same name, also from Apple.
Swift Cheat Sheet — A quick, high-level reference to Swift, from the creator of the Objective-C cheat sheet.
FAQ
Here we’ve answered some of the most frequently asked questions about Objective-C.
What is Objective-C?
Objective-C is a superset of the C programming language. It is mostly like C, but adds Smalltalk-like Object Oriented features.
What is Objective-C used for?
While it is possible to use Objective-C to write apps for any operating system or platform, the only mainstream use of Objective-C is for Apple Apps on their three operating systems: Mac OS X, iOS, WatchOS.
Do I have to use Objective-C for Apple apps?
No. There are two other options besides Objective-C:
Swift — The Swift programming language is the successor to Objective-C, supported by Apple for all new development of apps.
Compiling or running on top of Objective-C or Swift — There are a handful of ways to build non-native apps that work in the Apple environment. For example:
Is Objective-C dead?
No. Objective-C is still a worthwhile language to learn, for at least a few reasons:
At present, Swift’s Cocoa APIs are not fully mature. For some period of time, Objective-C will continue to be the only way to take full advantage of the Cocoa platform.
Additionally, the foundations for Cocoa are written in Objective-C. This is unlikely to change any time soon, even after Swift is fully mature. If you want to write complex applications that require an deep understanding of the platform, you’ll need Objective-C.
Until recently, all Apple apps were written in Objective-C, and many continue to be. This means that if you are tasked with maintaining, expanding, or enhancing these existing apps, you’ll need to know Objective-C.
How is Objective-C different than C?
Objective-C include all of C, and then adds Object Oriented principles to it, using Smalltalk’s syntax.
How is Objective-C different than C++?
C++ and Objective-C are both distinct solutions to the problem of adding Object Orientation to the C programming language.
Objective-C used Smalltalk as the model for how to implement Object Orientation, while C++ used Simula.
Can I use Objective-C in non-Apple systems?
Yes. Objective-C can be used anywhere, as long as the requisite runtime is installed on the system.
However, it is not very common to do this, and there are usually better (more fully supported) ways of writing applications for other platforms.
Perhaps the most compelling reason to use Objective-C in a non-Apple environment would be when porting an Apple App to Windows or Linux. In this case, you would need to also replicate the Cocoa Framework, which you can do with GNUstep.
Comments