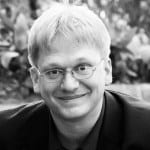
PHP Programming: Expert or Novice, There’s a Tutorial for PHP Devs of Any Skill Level
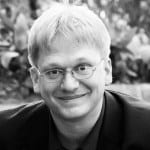
Disclosure: Your support helps keep the site running! We earn a referral fee for some of the services we recommend on this page. Learn more
PHP is the most popular programming language for server-side web development. It was originally conceived in 1995 as a fairly simple way to create dynamic HTML templates. But in the decades since it has grown into a powerful language, used for everything from popular blogging software to giant enterprise applications. Wikipedia used PHP. Even Facebook uses PHP (well, sort of).
One of the best things about PHP is that it is relatively easy to learn. Most beginners find the language straight-forward. Plus, the prevalence of extensible PHP-based apps (like WordPress and Drupal) make it trivial to find interesting projects to work on. The language itself, plus the ecosystem surrounding it, make it an ideal first language.
Tutorials
The best way to get started learning PHP is to just pick one of the many excellent, free tutorials on the language and dive right in.
Tutorials for PHP Beginners
These tutorials will get you started learning PHP. But don’t stay here too long. Many new PHP programmers go through many beginner tutorials. Just pick a couple to work through, and then move on to the intermediate and advanced material. And don’t forget to work on some real projects along the way.
- PHP Tutorial from W3Schools provides a good, general introduction to the language and basic syntax; their innovative “Show PHP” tool lets you work in-browser without having to run PHP scripts locally — great for absolute beginners;
- Tutorials Point has an entry-level PHP tutorial that is very similar to the one from W3Schools — check out the first few pages of each to see which one appeals to you more;
- A Simple Tutorial, included in the PHP documentation, is required reading for people just getting started;
- PHP 101 for the Absolute Beginner was created by Zend, one of the most important companies in the PHP community;
- YouTuber TeachMeComputer has a 25-part video tutorial on PHP
- Learning PHP in 30 Minutes is good if you don’t have the patience for 25 videos;
- Learn PHP in 15 Minutes if you don’t even have 30 minutes;
- PHP: Building Dynamic Websites, from Harvard Open Courseware provides a more academic approach to PHP, for people with some computer programming experience; the whole video series, which covers an entire course on dynamic web design, is worth watching.
- PHP Security Primer our own guide and reference cheat sheet.
Intermediate and Advanced Tutorials
These cover advanced PHP concepts in general, or touch on specific advanced topics and problems domains.
- Object Oriented PHP is a great video introducing object oriented programming concepts in PHP;
- Learn Advanced PHP Programming is a highly-rated, premium PHP tutorial from Udemy, with 52 lectures and 6 hours of video;
- Advanced PHP Programming is a 9-part video series; the creator of this series also has videos on several other advanced PHP topics;
- PHP Rocks has a series of intermediate and advanced tutorials;
- Advanced Object-Oriented Programming in PHP provides a number of excellent tips on writing classes in PHP;
- Advanced PHP is a three-hour video series on advanced topics;
- Build a CMS with PHP is a 126-part video series diving into a practical project, covering most of the common issues in PHP development;
- Advanced OOP PHP Tutorial is another video series, covering object-oriented PHP;
- Intermediate OOP in PHP, a 45-minute talk recorded at a PHP user group.
PHP Reference Material
- The Official PHP Documentation should be bookmarked by every PHP developer — you’ll end up here again and again;
- PHP Classes is a library of PHP classes which you can use or learn from;
- PHP Function Reference is a Mac OS X dashboard widget that provides offline access to the PHP function documentation;
- Awesome PHP is a curated list of the best PHP tools.
PHP Development Tools
- PEAR — PHP Extension and Application Repository is a package library for PHP modules, apps, and libraries written in PHP;
- PECL is a repository for PHP extensions written in C — a sister project to PEAR;
- Composer is a dependency management system for PHP;
- PHP Debug Bar puts a handy admin bar a the bottom of your web pages, providing on-page information about performance, errors, warnings, and custom data;
- Pinba is a PHP and MySQL monitoring tool for gauging the speed and performance of queries in your application; also check out Intaro Pinboard, which provides aggregates and displays data from Pinba;
- PHPMyAdmin is a MySQL administration tool written in PHP;
- PHP Beautifier reformats and beautifies PHP source files, fixing indentation and other style issues;
- PhpDox is a PHP documentation generator; phpDocumentor is a similar tool;
- PHPUnit is a unit testing suite for PHP applications;
- PHP Debug is a very helpful debugging tool that prints out a program trace, a list of all variables, included files, and processing times at the bottom of PHP-generated HTML pages;
- Faker can be used to provide fake testing data to your PHP app;
Frameworks
There are a lot of PHP application development frameworks, and most of them have converged on a similar set of features and architectural patterns. Here are the most notable and popular:
- Zend Framework is the premier, enterprise-level PHP framework;
- Laravel is a “framework for web artisans” with a focus on the developer experience;
- Symfony is a collection of framework components that can be used as a standalone development framework, or as the basis of other tools — Laravel is even built on top of Symfony;
- CodeIgniter is one of the oldest PHP frameworks; it has a focus on being light-weight and easy to use;
- Slim is a PHP “microframework,” designed to help developers write apps and APIs quickly;
- CakePHP is another older PHP framework (first release, 2005); it is designed to be “batteries included” — everything you need is baked in;
- Phalcon is a unique PHP framework, built as a C extension to PHP rather than as an in-language application, making it the fastest PHP framework available.
Also, depending on your application needs, it isn’t uncommon to treat the more robust content management systems (especially Drupal and WordPress) as application frameworks. This is only recommended if your application is content-focused.
Content Management Systems
There are even more PHP CMSs than there are frameworks, but only a handful are really worth using. The “big three” content management systems for PHP are:
- WordPress is the most popular blogging and content management application in the world, powering over 25% of world’s websites;
- Drupal is an advanced content management system with a modular architecture, built for extensibility;
- Joomla! is about half-way in complexity (and popularity) between WordPress and Drupal;
Other notable CMSs are:
- MediaWiki is the most well-known wiki application; it powers Wikipedia, and many other popular wiki sites;
- Zikula is a CMS and application framework built on Symfony;
- BigTree is a CMS built by and for designers, with a focus on the user experience;
- October CMS is a relatively new PHP CMS, built on Laravel;
- Tiki is a weird and wonderful PHP CMS that includes all the features;
- Pico is a flat-file CMS, meaning that content is stored in files rather than a database — the perfect solution for simple sites and single-author blogs, without all the overhead of WordPress or Drupal;
Libraries and Modules
- PHP-GTK provides PHP bindings to the GTK+ user interface library, allowing for rapid development of desktop applications;
- TCPDF is a library for working with PDFs in PHP applications;
- HTML Purifier scrubs and validates HTML5 output, removing cross-site scripting security vulnerabilities and making the markup compliant with the HTML5 standard;
- WideImage is a popular image manipulation library for PHP apps;
- PHP-CPP is a C++ library for building PHP extensions in C++.
Templating Systems
- Smarty is one of the oldest template systems for PHP;
- Dwoo is a Smarty-compliant templating system that works with several PHP frameworks;
- Foil is a templating engine deigned to use native PHP, rather than a specially-designed templating language;
- Lex is a lightweight template parser;
- Mustache.php is a PHP implementation of the Mustache template language.
Editors and IDEs
- VS.php is a PHP IDE for Microsoft Visual Studio;
- Zend Studio is a PHP IDE from Zend, makers of the Zend Frameworks, and major sponsors of PHP language development;
- PHP Designer is a PHP IDE with built-in support for HTML, CSS, and JavaScript;
- NuSphere PhpED is another popular PHP IDE;
Books
PHP is so popular today that you can get pretty far in your learning with online tutorials and videos. However, there are also a number of great books on PHP, many of which cover material that you can’t easily find online.
Books for PHP Beginners
- Learning PHP, MySQL & JavaScript: With jQuery, CSS & HTML5, by Robin Nixon, is a best-selling introduction to programming in PHP, along with the rest of the languages most critical for web applications development — the perfect guide for those just getting started;
- PhP: Learn PHP Programming Quick & Easy, by Troy Dimes, is a simple, step-by-step introduction to basic PHP programming;
- The Joy of PHP: A Beginner’s Guide to Programming Interactive Web Applications with PHP and MySQL, by Alan Forbes, is a fun and practical introduction to PHP programming;
- Learning PHP: A Gentle Introduction to the Web’s Most Popular Language, by David Sklar, is an up-to-date resource for learning PHP, including information on PHP 7;
- PHP: Learn PHP in 24 Hours or Less — a Beginner’s Guide to Learning PHP Programming Now, by Robert Dwight, is a fast introduction for people who need to learn the basics of PHP right away;
- Programming PHP, by Tatroe, MacIntyre, and Lerdorf, is one of the best, and most complete, introductory texts on PHP; one of the authors (Rasmus Lerdorf) was the inventor of the language;
Intermediate and Advanced PHP Books
- Modern PHP: New Features and Good Practices, by Josh Lockhart; PHP has a reputation for poorly written code, mostly because of outdated practices and inexperienced developers; this book will help you understand how to write high-quality PHP code according to today’s recognized best-practices;
- PHP Cookbook: Solutions & Examples for PHP Programmers, by Sklar and Trachtenberg, is a good reference book for expanding your programming toolkit in PHP;
- PHP Objects, Patterns, and Practice, by Matt Zandstra, introduces PHP’s object-oriented features and illustrates a number of PHP design patterns;
- Learning PHP Design Patterns, by William Sanders, explains classic object-oriented design patterns and how they can be implemented in PHP.
Special Topics in PHP Programming
- PHP Web Services: APIs for the Modern Web, by Lorna Jane Mitchell;
- Essential PHP Security, by Chris Shiflett;
- Extending and Embedding PHP, by Sara Golemon.
PHP Today
The PHP language, and applications built with it, revolutionized the web. PHP made it easier than ever before to build and deploy dynamic websites and web-facing applications. It sparked the growth of the shared hosting industry, and shaped the way these plans were designed and sold.
Today, a new revolution is underfoot. Between the rise of JavaScript-based frameworks and the constant criticism of PHP’s flaws, it can seem to some new developers that PHP isn’t worth their time. Nothing could be further from the truth.
PHP powers a majority of the web, a situation unlikely to change rapidly in the near future. For every cool new app built in Node.js, hundreds of PHP-based sites are launched. WordPress alone represents a huge economy unto itself.
PHP is, and will remain, a highly valuable skill.
Comments