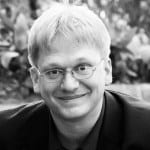
Get Started Designing Circuits With Verilog
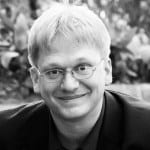
Disclosure: Your support helps keep the site running! We earn a referral fee for some of the services we recommend on this page. Learn more
Verilog is a hardware description language (HDL). This is similar to a programming language, but not quite the same thing. Whereas a programming language is used to build software, a hardware description language is used to describe the behavior of digital logic circuits. That is to say, an HDL is used to design computer chips: processors, CPUs, motherboards, and similar digital circuitry.
Verilog History
Verilog was one of the first modern HDLs. There were several earlier HDLs, going back to the 1960s, but they were relatively limited. Until Verilog (and its close competitor, VHDL), most circuit design was done primarily by hand, translating the behavior specified in a formal Hardware Description Language into drafted circuit-board blueprints.
Verilog started in the early 1980s as a proprietary (closed source) language for simulating hardware — in part for doing hardware verification work. (The name is a combination of “verification” and “logic.”) The design incorporated ideas from other HDLs (mostly HiLo) and also from programming languages (mostly C). Once people started using the language, it was clear that it could be used to design new hardware. This required designing hardware synthesis tools which could translate the logic of an HDL module into a physical design.
In 1990, the company that owned Verilog (Cadence) decided to open source the language. They transferred the rights to a new non-profit organization called Open Verilog International (which later merged with the equivalent organization for VHDL to form Accellera). Hardware vendors quickly began modifying and extending Verilog for their own purposes, creating dozens of slightly-incompatible versions. OVI asked the IEEEto standardize the language, which it did in 1995. IEEE continues to be the authoritative standards body for the Verilog language, and Accellera is the primary driver of language development.
Verilog has changed a great deal over the last three decades. Prior to standardization, each new version from Cadence introduced a large number of new features. Since the standard was taken up by the IEEE, there have been three language specifications — the latest in 2005.
The Verilog Family
In addition to the core Verilog language, there are two major members of the Verilog family.
- SystemVerilogis a superset of Verilog which adds a complete hardware verification language.
- Verilog-AMS is a derivative of Verilog that adds features for describing analog and mixed-signal systems.
Verilog Syntax and Examples
The syntax and structure of Verilog is very similar to C. The major difference being that it includes structures and operators for describing hardware-specific details.
Some things are made more explicit in Verilog — propagation time and signal strength, for example — which aren’t really dealt with in languages like C.
Other things are actually abstracted a bit — like flip-flops (exchanging the values of two variables) which can be shown in Verilog without the need for temporary assignment to ad-hoc variables. In the following example, the values in the x and y variables are swapped:
begin
x <= y;
y <= x;
end
To give you a little feel for the language, here are three examples of the same multiplex process. These all combine the signals from two inputs into a single output.
Example: Continuous Assignment
wire out;
assign out = sel ? a : b;
Example: Procedure
reg out;
always @(a or b or sel)
begin
case(sel)
1'b0: out = b;
1'b1: out = a;
endcase
end
Example: If-Else
reg out;
always @(a or b or sel)
if (sel)
out = a;
else
out = b;
The always
keyword forms a never-ending loop. When the @()
operator is added, one iteration of the loop happens whenever the named values change.
Verilog Resources
Because Verilog is not a traditional software programming language, resources are more focused on books. But we have put together some of the best online resources as well.
Online Resources
- Tutorials
- Verilog Tutorial from Asic World: the information is excellent, especially for those who need an entry-level tutorial. But you will have to ignore its terrible design.
- Verilog Primer: a bare-bones four chapter online book that tries to deliver “just the facts” on Verilog. Very good for getting yourself unconfused.
- Introduction to Verilog: a free nine-chapter course.
- Tools
- VeriPool: free software tools for Verilog development.
Books
Unlike popular software development languages, there just aren’t that many online resources and tutorials for Verilog. If you want to learn the language, you’ll need to acquire some physical books.
Introductory Books
These are for people just starting to learn Verilog.
- Introduction to Verilog: one of the better “just getting started” books available.
- Fundamentals of Digital Logic with Verilog Design: an introduction to the topic, written and designed as a textbook for a college-level course. Integrates CAD (computer-aided design) software for translating Verilog into physical circuit design, and documents how real chips actually work.
- Verilog by Example: A Concise Introduction for FPGA Design: FPGA is Field Programmable Gate Array, a type of integrated circuit which can be programmed after manufacturing (and reprogrammed). This combines the speed of direct hardware implementation (as in an ASIC) with the flexibility of software programming. Verilog can be used to program FPGAs (in fact, if you are just getting started, you are much more likely to be able to implement your designs on an FPGA than an ASIC, which would have to be custom manufactured). This book provides a solid introduction to this topic.
- Digital Design (Verilog): An Embedded Systems Approach Using Verilog: another college-course textbook with introductory information on Verilog, this one with an emphasis on designing embedded systems (which is where you would most likely find yourself needing to design new FPGA images in the real world).
- Digital Design: With an Introduction to the Verilog HDL
- Digital Design and Verilog HDL Fundamentals
- Verilog HDL Synthesis, A Practical Primer
- SystemVerilog for Design Second Edition: A Guide to Using SystemVerilog for Hardware Design and Modeling
Intermediate and Advanced Books
Books for people who already know Verilog, and want to increase their skill level.
- Verilog and SystemVerilog Gotchas: 101 Common Coding Errors and How to Avoid Them: a highly recommended book for intermediate Verilog programmers looking to become experts.
- Verilog Designer’s Library: a sort of Verilog recipe book for working Verilog developers.
- Advanced Chip Design, Practical Examples in Verilog: one of the more recent additions to the Verilog canon, this 2013 book is an in-depth look at chip design from a master of the craft.
- Digital Logic RTL & Verilog Interview Questions
Books just about System Verilog
- The UVM Primer: A Step-by-Step Introduction to the Universal Verification Methodology
- SystemVerilog for Verification: A Guide to Learning the Testbench Language Features
- SystemVerilog Assertions and Functional Coverage: Guide to Language, Methodology and Applications
Alternatives to Verilog
Verilog is one of the two most commonly used hardware description languages. The other is VHDL. Additionally, C++can also be used as an HDL and HVL, though mostly only for high-level behavioral design. Most serious hardware developers are fluent in all three, plus major low-level operating system languages like C.
Comments