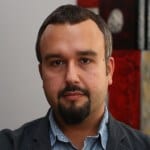
Learn WSGI, and Let Python Sweat the Small Stuff
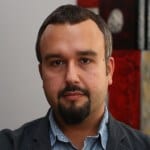
Disclosure: Your support helps keep the site running! We earn a referral fee for some of the services we recommend on this page. Learn more
Back in the day, developing web applications in Python used to be problematic, because developers had to take special care and assure that their web application would smoothly run on different web servers and various web frameworks for Python. The choice of a specific Python web framework during application development limited the choice of compatible web servers, capable of running the finished application.
Web Server Gateway Interface (WSGI) was introduced as the solution to this problem. WSGI is a specification for a standardized interface for communication between web servers and Python web frameworks or applications. If an application, or a framework, is written to comply with the WSGI specification, then it will run on any web server supporting that same specification.
Of course, Python is not the only programming language with a standardized interface specification. Many modern programming languages use the same approach, so for example, Ruby uses its own Rack server interface, JavaScript relies on its JSGI gateway interface, while Perl uses PSGI.
Brief History
The WSGI specification was originally introduced in the Python Enhancement Proposal 333 (PEP 333), written by Phillip J Eby and published in December 2003. This initial WSGI spec draft laid out the basic principles and goals for WSGI — it had to be easy to implement, simple and universal, and had to facilitate easy interconnection of existing servers and frameworks.
WSGI was quickly adopted by Python server and framework authors and developers, and became the de facto standard for Python web application development. The latest version of the WSGI specification is V1.0.1, published in PEP 3333 on September 26, 2010.
WSGI Specification Overview
The WSGI specification declares three specific roles: the server side, the application side, and the middleware component, which implements both server and application sides of the interface.
The Application Side
The application side of the WSGI is a simple object that accepts two arguments and can be called from code. This example shows a simple WSGI application that returns a static “Hello world!” page:
def simple_app(environ, start_response): status = '200 OK' response_headers = [('Content-type','text/plain')] start_response(status, response_headers) return ['Hello world!n']
Application side objects can be invoked multiple times, as all servers make such repeated requests.
The Server Side
The server side of WSGI simply receives requests from the HTTP client, invokes the application once for each request, and sends the response returned by the application to the client.
The Middleware Component
Middleware components defined in the WSGI specification use both sides of the interface — the application side, as well as server side. Middleware functions are transparent to both the server side and the application side.
Middleware components usually preform functions like routing requests to different application objects based on the target URL, allowing multiple applications to run side by side in the same process. They enable load balancing, remote processing or content post-processing.
This example shows a simple middleware component that uppercases everything it receives:
class Upperware: def __init__(self, app): self.wrapped_app = app def __call__(self, environ, start_response): for data in self.wrapped_app(environ, start_response): return data.upper()
Using WSGI
WSGI is now accepted as a standard for Python web application development. Python version 2.5 and subsequent releases feature embedded WSGI support. In Python versions 2.4 and earlier, WSGI support can be installed separately. An updated version of the WSGI specification declared in PEP 3333 is available for Python 3.
If you are developing web applications in Python, simply use an industry proven standard, like Django, Flask, or Bottle Python web frameworks, or any other current Python framework. It is not necessary to learn much about the WSGI specification to build applications. Just use any current generation web application framework and you should be in the clear, as they all support WSGI.
On the other hand, if you are developing a new web application framework yourself, then you should definitely take a good look at the WSGI specification and check out some learning resources for WSGI.
WSGI Resources
If you need to find out more about WSGI, we suggest taking a look at some of the following online resources:
- The WSGI Community Site is obviously the first place to visit. You can find many useful resources, and there is also a nice Learn About WSGI section. This site also lists the frameworks and servers that support WSGI.
- You can also read the full Python Enhancement Proposal 333 and the Python Enhancement Proposal 3333 for more information.
- Pylons Web Development Framework Online Documentation has a very nice and detailed section on WSGI.
- Codepoint’s WSGI Tutorial will demonstrate the WSGI specification at work using a few simple examples.
WSGI Books
Books that specifically cover WSGI are hard to come by, and you will usually find chapters dealing with WSGI in various Python web application programming books, like this one:
- Foundations of Python Network Programming: The Comprehensive Guide to Building Network Ppplications with Python by Goerzen, Bower, and Rhodes: The chapter on web application programming covers both the WSGI standard for component interoperability, as well as modern web frameworks like Django.
Conclusion
WSGI is a vital Python specification, but in reality, most Python developers needn’t worry about it. As long as they are using a major framework, they’ll benefit from WSGI without giving it a second thought. In other words, most developers don’t require an intimate knowledge of WSGI, because the specification has been implemented in all major Python frameworks.
That said, WSGI is still relevant, although it is not a prerequisite for Python programming. One notable exception involves software engineers who decide to work on Python frameworks rather than Python applications. As far as they are concerned, WSGI is an indispensable spec, and they have to know the ins and outs of WSGI if they hope to integrate it in their framework.
This is obviously a (very) tight niche, but that does not mean WSGI development has ground to a halt. It merely means the community is not big, but it’s there.
Further Reading and Resources
We have more guides, tutorials, and infographics related to coding and development:
- Python Introduction, Resources, and FAQs: if you are new to Python, this introduction will get you going.
- MPI — Introduction, History and Resources: the Message Passing Interface (MPI) allows programs and other computer systems to send messages to each other.
- Linux Programming Introduction and Resources: this deep dive into Linux programming gets down into the kernel where all the action is.
What Code Should You Learn?
Confused about what programming language you should learn to code in? Check out our infographic, What Code Should You Learn? It not only discusses different aspects of the languages, it answers important questions such as, “How much money will I make programming Java for a living?”
Comments