
What Is Event-Driven Architecture?
Event driven architecture is a way of orchestrating the way software components interact with each other and their environment.
Non-Event Driven Coding
In a conventional, non-event-driven approach, if you want one action to cause another action, you usually would have to write an explicit connection between those two actions. This had to be done inside the causal agent.
In other words: if I want A to trigger B, I have to add some code to A.
Event-Driven Coding
Event-driven architecture abstracts this to an environment that contains triggers and listeners.
You can add event triggers to any action (even if you do not know yet that it will be meaningful later) and add listeners to components (even if there is nothing yet to trigger them). Listeners wait for triggers and then launch an action.
Event-Driven Coding for UI
Event-driven programming is typically used in user-interface situations: The system listens for events such as mouse-clicks or keystrokes.
JavaScript already had easy support for event-driven architecture because of its in-browser event-listening usage.
Node.js took advantage of this built-in characteristic to create an event-driven development framework for creating applications.
Non-Blocking I/O Operations and Single Threading
In Node.JS, I/O operations do not block other I/O operations, allowing multiple connections to be made simultaneously without disruption.
Communications Operate on a Single Thread
All connections operate in a single thread, so there is no performance lag between multiple concurrent processes.
Concurrent Applications
Because of these characteristics, Node.js is highly useful for highly-concurrent, real-time applications, such as gaming.
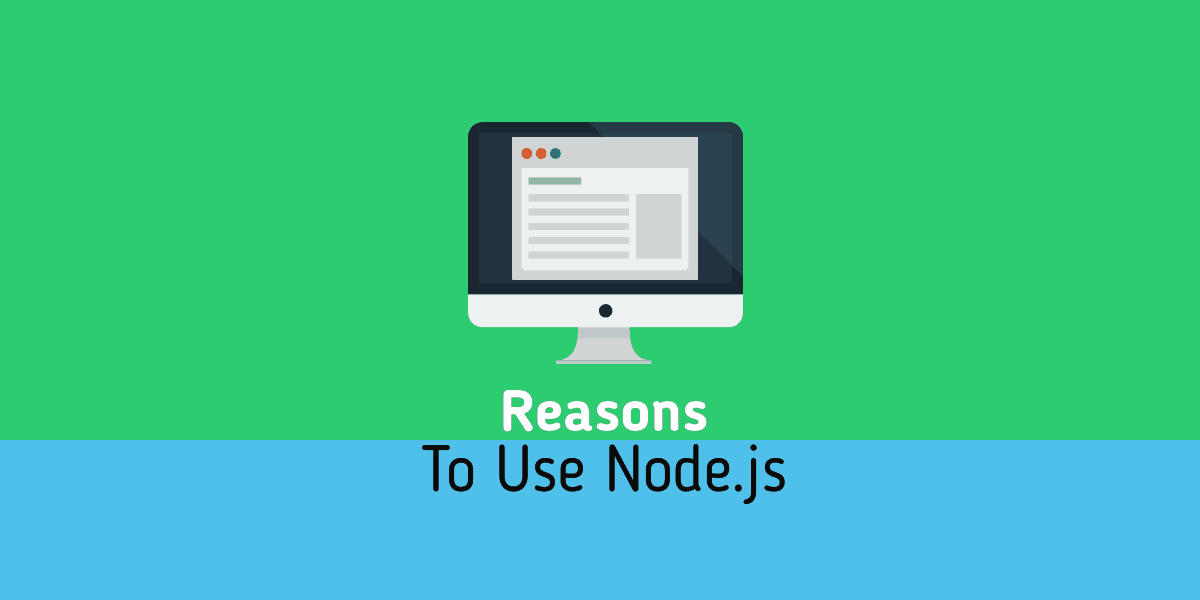
Top Features of Node.js 12
In April 2019, the most recent major release of Node.js (version 12) was shipped. Some of the updates and upgrades included in this release include:
- An updated version of the V8 JavaScript engine, offering faster execution of JavaScript code, improved memory management, and extended support for ECMAScript syntax
- Support for private class fields, which restricts where code variables can be accessed
- Improved performance via caching
- Use of an optimized compiler
- Security enhancements
- Diagnostic reports that allow developers to create reports when certain events occur
Reasons to use Node.js
Here are some reasons to use Node.js:
- Huge Talent Pool
- Speed
- Node Package Manager
- Language Simplicity
- Multiple Connection Support.
Huge Talent Pool
The fact that there is a huge talent pool helps you in a couple of ways
Given that there are many talented node.js developers, you can easily find one if you want to outsource the job.
On the other hand, if you plan to do the coding yourself, you’ll find a lot of mpde.js developers who are eager to answer your questions. (Programmers are like that.)
Speed
The fact that Node.js is a very fast language is no secret.
Since it is built on Google’s V8 JavaScript engine you know that you can depend on it and that it will only get faster over time.
Node Package Manager
If you want to be an efficient coder, you need to learn to use the code that others have already created.
The Node Package Manager (NPM) is the world’s largest source of open-source libraries. It makes installations a piece of cake.
Language Simplicity
Often complex projects require using several different languages like C, Assembly, and PHP. Node.js is not that way.
With JavaScript/Node.js, you can write an entire application from client to the server to database in one programming language. And that’s a huge help.
Multiple Connection Support
If you are going to be writing a gaming or chat application, Node.js is the language for you.
This is thanks to the event loop and its support for multiple, concurrent connections.
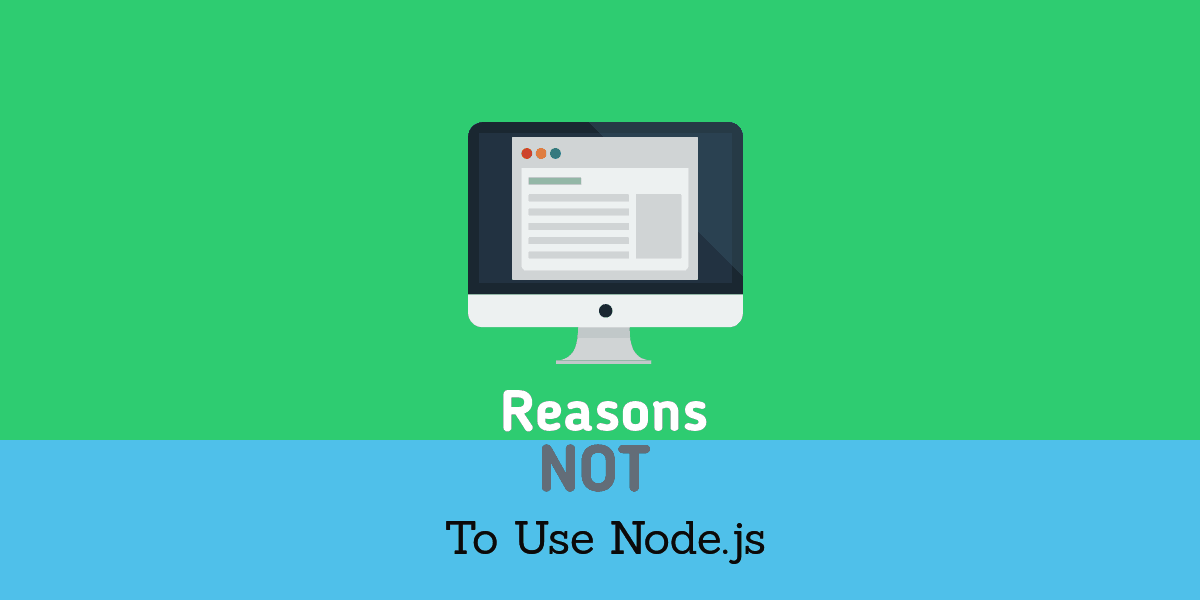
Reasons Not to Use Node.js
Node.js is not a “fix-all” development framework. There are certainly some applications where it is not the best option.
Here are some reasons not to use Node.js:
- Heavy data computation and processing
- SQL support
- Incompatibility issues
- API changes frequently
- Immaturity of some tools in the npm registry
- Price (hosting)
Heavy Data Computation and Processing
Node.js is really fast at handling I/O. Unfortunately, it is designed to do one thing at a time (though the asynchronous, non-blocking nature means that there aren’t many slowdowns).
As a result, it is not very efficient for heavy data computation and processing. Furthermore, Node.js is lagging in terms of data science and other helpful packages.
SQL Support
Node.js has not yet implemented fully mature SQL implementation.
So if your application is going to be very database intensive, you might want to use a different language — at least for the time being.
Incompatibility Issues
If you are working with legacy tools such as old libraries, you may want to avoid Node.js. It has not been developed for this purpose, but this may change in the future.
Backward Compatibility
Node.js is still being actively developed.
Many of the changes are not backward-compatible. And this can be a major issue if you don’t want to spend a lot of time maintaining old code.
Price
Node.js hosting options are often more costly than alternatives. Support for Node.js isn’t as common as other languages, and your application may require a more expensive hosting option (e.g., VPS hosting).
So if PHP or another server-side language will work for your application, it may be best to go with it.
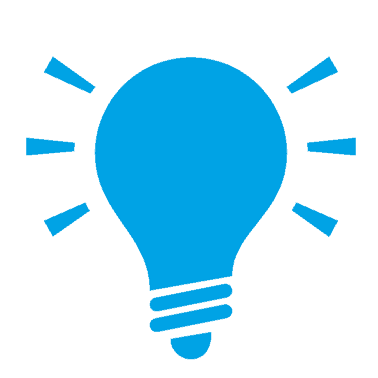
Looking for a great deal on Node.js hosting?
A2 Hosting has VPS plans optimized for Node.js. And this host scored #1 in our recent speed and performance tests. Right now you can save up to 50% on A2 plans. Use this discount link and get a free site migration too.
Major Features of Node.js
Here are the 8 features that stand out to most people.
Plan | Transfer |
---|---|
Professionally managed administration | Administrator takes care of basic server administration tasks |
Other programming languages like PHP, Perl or Python | Can be used for creating dynamic websites |
Database (usually MySQL) | Handles data storage |
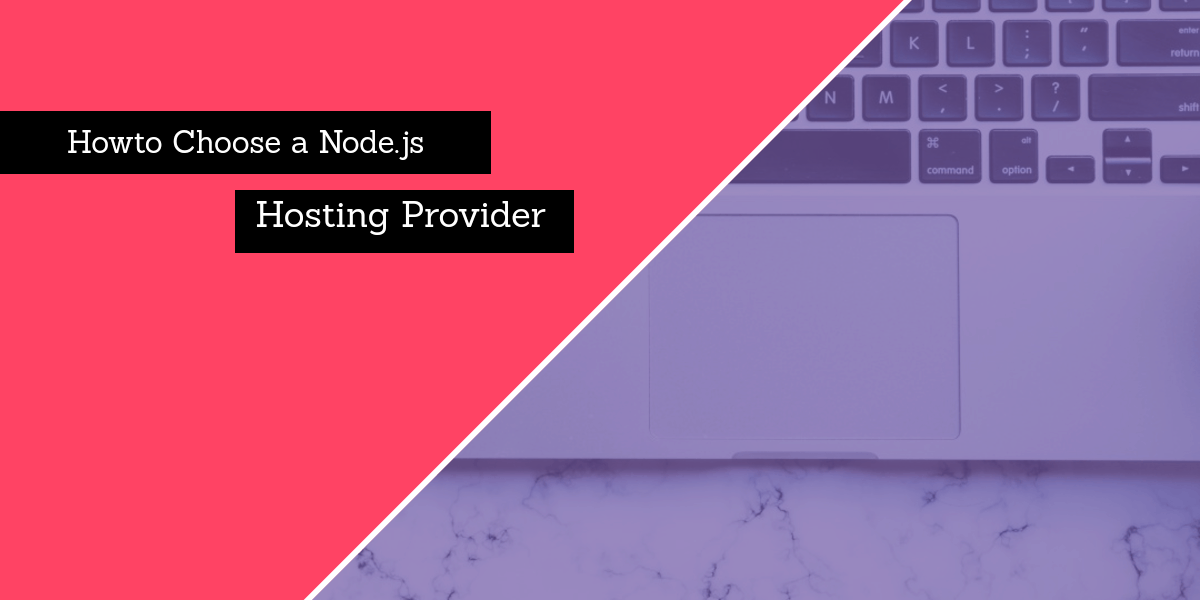
How to Choose a Node.js Hosting Provider
Node.js is still relatively new, so most web hosts do not support it yet.
On the other hand, it is also very trendy, so there are a number of specialized web hosting providers that are well-optimized for Node.js applications.
Do Your Homework
Be sure to compare hosting options in detail before committing to one.
More Than the Usual Features
You want to look past the usual considerations when selecting a web host provider — things such as resource allocation and the inclusion of bonus features.
There are other things you need to keep in mind when selecting the option that is the best for your needs.
Factors to Consider
Here are some of the factors you should consider:
- Which programming languages does the host support
- Node.js, as a more developer-oriented language, ensure that the user-friendliness of your host matches your skillset
- Support for Node.js tends to be offered only on Virtual Private Servers (VPS) plans or dedicated servers which may be a problem with a lower web hosting budget
- Are other alternatives such as PHP more suited to your needs
- Does your host of choice have a good reputation and reliable uptime figures?
With that said, what are some specifics that you should consider when looking for a Node.js hosting plan? Well, we think the following are important:
- Node.js versions supported
- Commitment to Node.js and Availability of APIs / Developer-Oriented Tools
- Technical Support and Customer Service
- Pricing and cost-effectiveness
- Hosting company reputation and reliability.
How Long Will Node.js Version Support Last?
Major releases of Node.js ship every six months, with even-numbered versions shipping in April and odd-numbered versions shipping in October.
Whenever an odd-numbered version ships, the most recent even-numbered version transitions to the status of Long-Term Support (LTS), which means the version gets active support for the next 18 months. Afterward, it will get 12 months of maintenance support.
How to Upgrade Appropriately
Actively supported versions receive non-breaking backports of changes a couple of weeks after the current version receives them, while maintenance releases only include critical fixes and the applicable documentation updates.
What this means is that the version you are using will get some type of support for the next two and a half years or so It is up to you to upgrade your applications appropriately.
Upgrades on a VPS or Shared Hosting Plan
When it comes to web hosting, you will need your provider to update its environment as well.
Obviously, this is not a problem if you have purchased a dedicated server (since you have full control over your environment), but for those with VPS or shared hosting plans, it is important to check on the hosts’ upgrade policy before signing on the dotted line.
Specific Requirements When Using Node.js
In addition, to support for Node.js, you will want to see what the host offers in terms of developer tools.
The specifics will vary from user to user, so it is best to take stock of what you need and then find a host that meets your requirements.
Using MySQL Databases and Other Technologies
For example, many hosts offer MySQL databases and SQL Server instances, but what if you are looking for MongoDB so that you can implement a full-stack JavaScript application?
Can you use Redis, a NoSQL data store?
Can you easily integrate with Heroku for things like application build previews, GitHub for version control, or Jenkins for continuous integration?
Does the host offer WebSocket support or offer load balancing?
These are all questions you may want to ask.
Hosting Companies that Prioritize Node.js
Essentially, you must consider whether your host treats Node.js as a first-class citizen or not.
We do not think you should completely disregard a provider if it doesn’t prioritize Node.js, but we do think you should do your research to ensure that support goes further than “we know you can install Node.js and run your app.”
The web host gets bonus points if it has experience integrating Node.js apps with things like Git and Heroku for continuous deployment or with cloud-vendors.
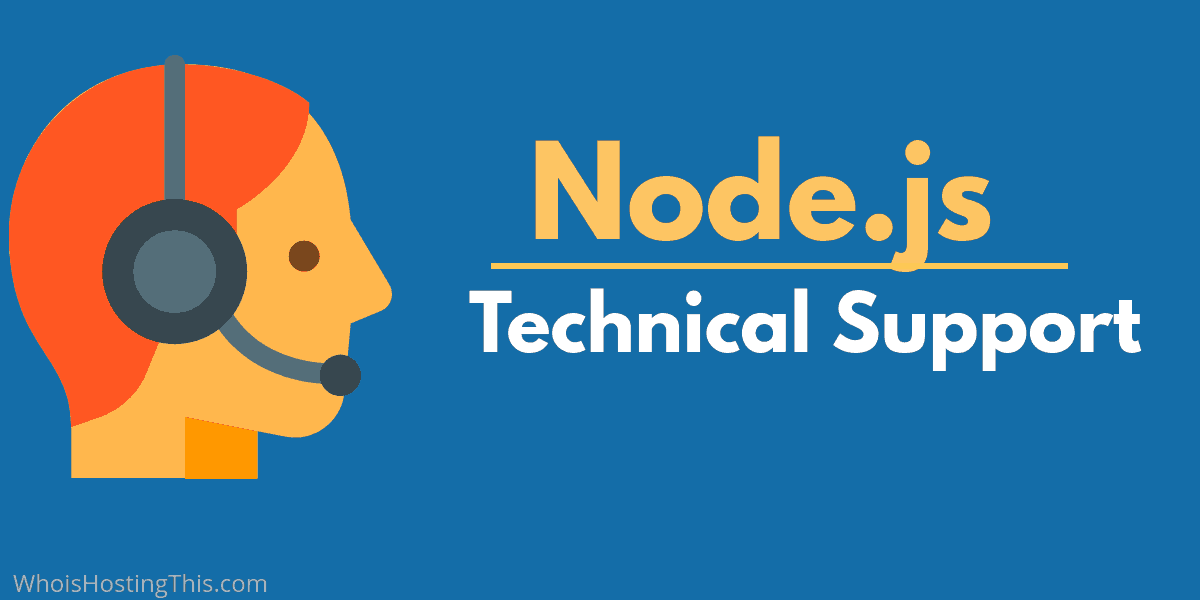
Technical Support and Customer Service
There are a lot of web hosting providers whose software supports Node.js, but they then state explicitly that all Node.js code and related issues are unsupported.
In some cases, this may be okay, especially if you have an in-house technical team. However, if you think you might need assistance in this area, be sure to check the fine print to see if you will get support for issues that pop up as a result of your Node.js use.
Great Places to Study Node.js
Perhaps you are interested in Node.js hosting but don’t yet have the skills to tackle Node.js head-on? If so, here are some great places to study Node.js online.
Method: | Best for: |
---|---|
Nodeschool.io | Intermediate Learners |
Rithm School | Beginners |
Colt Steele’s Advanced Web Developer Bootcamp (on Udemy) | Advanced Learners |
Alternative Options for Node.js Hosting
In addition to the more popular web hosting options, we suggest you consider players like Heroku, Microsoft Azure, Google Cloud Platform, Amazon Web Services and Digital Ocean when purchasing a Node.js hosting package.
There is also Nodejitsu, which is the leading pure-play Node.js hosting provider.
Most of these options are cloud-based providers, so by opting for one of these, you will get all the benefits typically associated with cloud-based hosting: easy scalability, redundancy, and support for failover.
Considerations When Choosing a Node.js Alternative
With the exception of Nodejitsu, such options tend to support multiple languages. If you need a “polyglot” web hosting service, this might be the way to go for you.
The caveats, however, are that these tend to be developer-oriented options and may come with a steep learning curve, as well as a larger hosting bill.
Cloud-based options typically cost more than more traditional hosting options.
Best Node.js Hosting
When selecting the best hosts, we stayed with and chose from a list consisting of more traditional web hosting providers.
Features to Look For
In addition, to support Node.js, hosts may offer:
- A free domain name
- Use of CDNs to improve website performance
- SSD (solid-state drive)storage
- Free SSL certificates
- Use of GUI control panels (e.g., cPanel) and other add-ons for your website
- Choice of data centers
- Uptime guarantees
- Money-back guarantee
Here are some good options if you are looking for Node.js web hosting:
A2 Hosting: Developer-Friendly
A2 Hosting: in addition to being an all-around solid host, A2 is one of the few providers offering support for Node.js (even with shared hosting plans).
A2 emphasizes the developer experience, so you are likely to find that they support whatever it is you need to use for your application.
If not, the A2 team encourages you to file a feature request for an addition to its development queue.
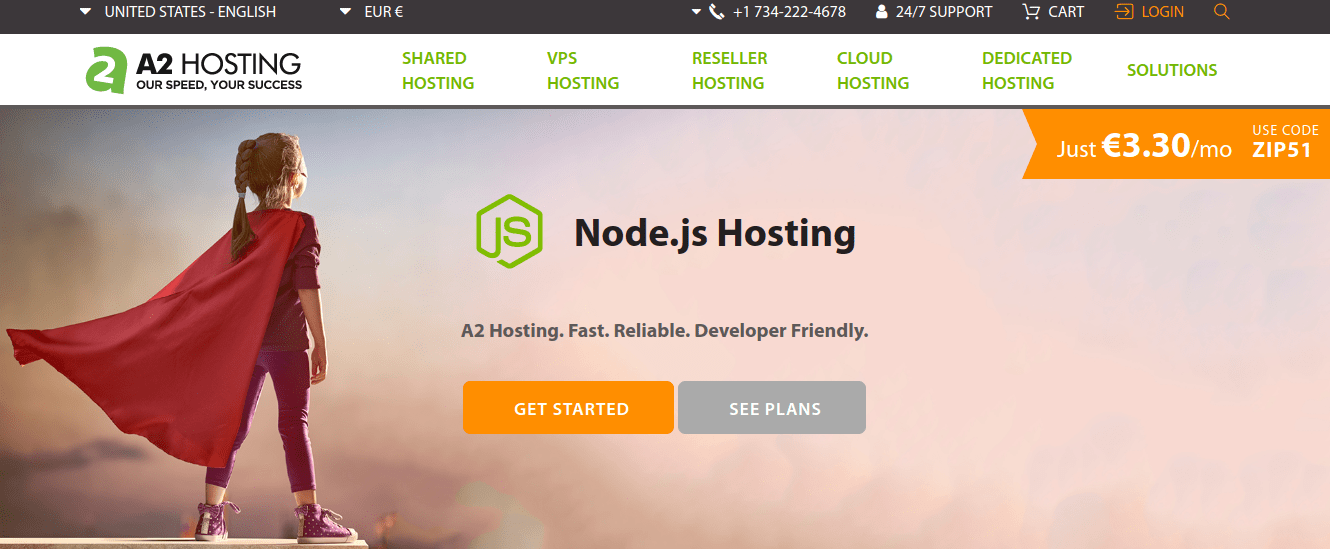
SiteGround: Quality Support
SiteGround is another great all-around web hosting provider, but support for Node.js is only offered on its dedicated server.
This limitation means that SiteGround is not different from any other provider that offers dedicated servers.
However, we still had to give a nod to SiteGround due to the fact that their web hosting offerings are competitive and are backed by quality customer service.
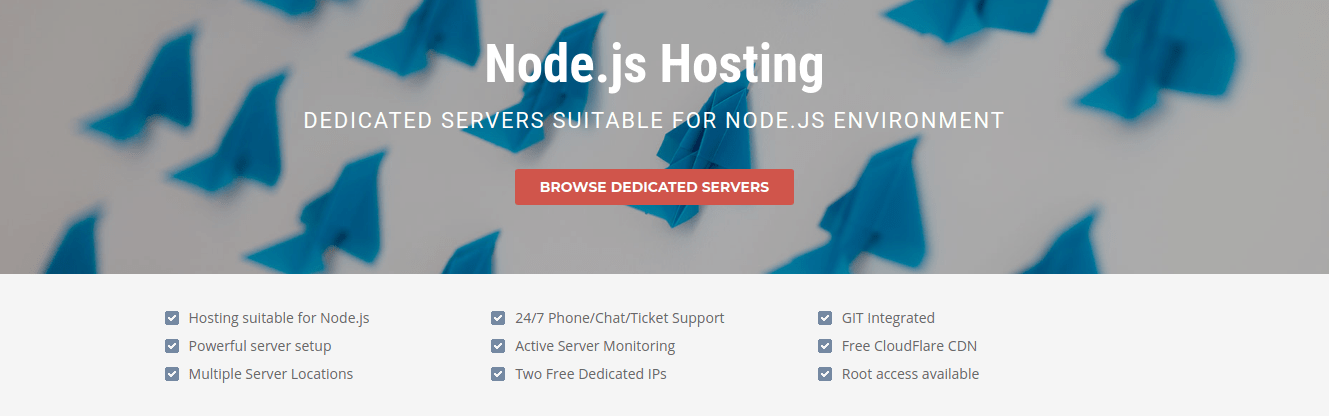
Liquid Web: High-Performance Hosting
Liquid Web offers high-end, advanced web hosting solutions that support Node.js.
Like SiteGround, Liquid Web offers solid options that are backed by top-notch customer service.
However, do note that most of the company’s options (both traditional and cloud-based) are managed, so if you are less technically-inclined or you want to outsource some (or all) of the management related to your web hosting while still purchasing a VPS plan or dedicated server, Liquid Web might be the best option for you.
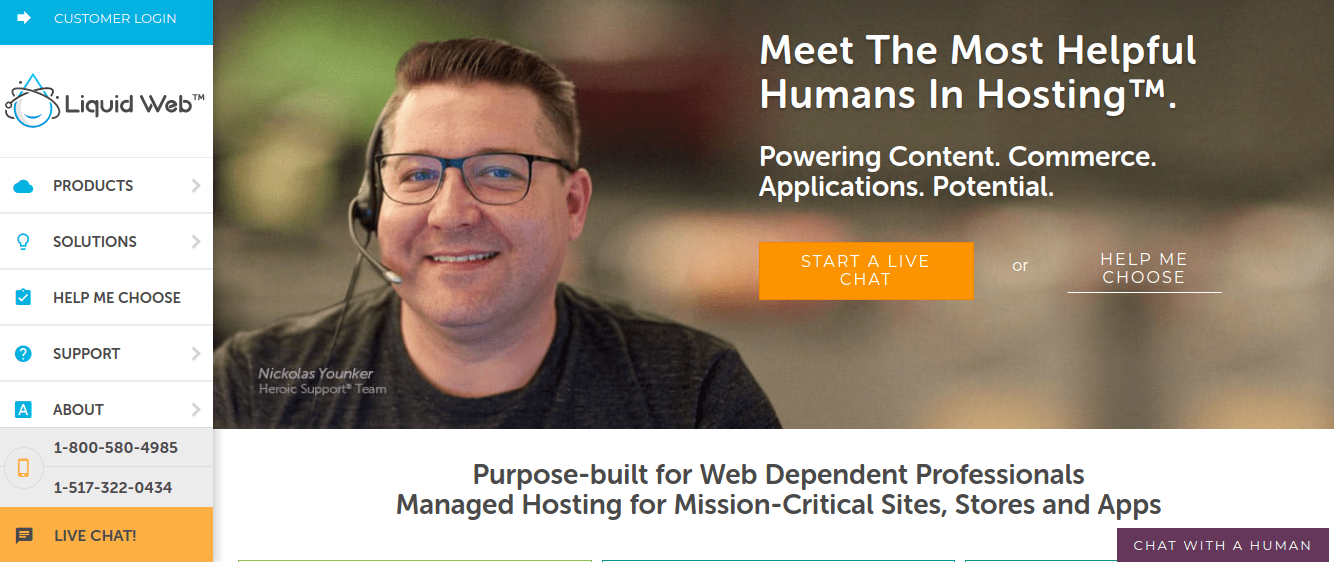
Node.js Hosting Summary
Node.js is an event-driven JavaScript runtime environment built on Chrome’s V8 JavaScript engine designed for speed and flexibility.
Node.js brings JavaScript to the back-end, allowing the rise of what are called “full-stack JavaScript applications.”
While there are plenty of upsides to using Node.js, it can be challenging to find a web hosting provider that supports full-stack JavaScript applications, especially if you are on a budget.
Regardless, there are certainly options, both traditional and cloud-based, even if you are more limited than if you had chosen, say PHP, instead.
Other features in Languages and Frameworks
- ASP.NET
- .NET Framework
- VB.NET
- Laravel
- PHP
- Ruby on Rails
- Perl
- Django
- Python
- Server Side Includes
- Java
- ColdFusion
- ASP
- CodeIgniter
- CakePHP
- Symfony
- PHP 5
- PHP 7
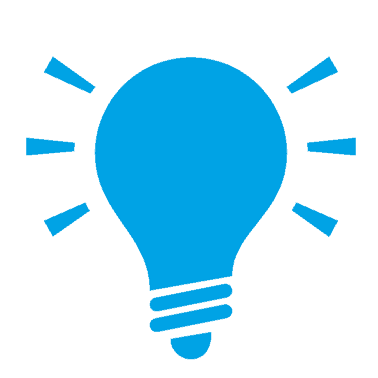
Looking for the right Node.js host?
A2 Hosting scored #1 in our recent speed and performance tests. They offer VPS plans optimized for Node.js. Right now you can save up to 50% on A2 plans. Use this discount link and get a free site migration too.
node.js Frequently Asked Questions
- Does cPanel support Node.js?
Yes, cPanel recently released support for Node.js, so you can use cPanel in environments you plan to host apps written using Node.js. In fact, cPanel’s Node.js Selector makes it easy to set up Node.js apps. Simply log in to your cPanel account and click on the “Setup Node.js App” icon. Then click “Create application” and follow the prompts.
- How do I run a Node.js application?
You can run Node.js applications locally by setting up a web server
Then run your web server using
node app.js
Next, navigate to http://localhost:3000 to view your app's output. Be sure to first install Node.js and the Node Package Manager.
- Who uses Node.js?
There are many developers and businesses that use Node.js. Top firms using Node.js include Netflix, LinkedIn, Uber, Trello, Walmart, and NASA. Node.js is a flexible tool that can be used in a variety of applications, which is partially why it is so popular.
- Why is Node.js a good choice for real-time web applications?
Node.js processes requests using an event-driven model. What that means is that Node.js handles a single event and sends it off to perform an action, then while it's waiting for the results of that action it can go ahead and handle additional events. That might not sound like a big deal, but in the traditional thread-based model once a server receives a request it doesn't do anything else until that request has been completely handled.
This means that Node.js can handle lots of requests far more efficiently than a thread-based model. That's just what you want and need if you have an application that takes in input from multiple users simultaneously and adjusts the experience of every user based on the collective input of multiple users.
- Can I build a content-management system with Node.js?
Yes, you can. However, there may be better options available for the task. The real power of Node.js is its ability to handle loads of inputs and outputs in a non-blocking fashion. A content-management application usually manages cacheable web pages, and non-blocking I/O generally isn't important to accomplish that task.
- What type of database can you use with Node.js?
You can use any type of database with Node.js as long as you can find a package with the necessary drivers to let Node.js interact with the database system. Thankfully, there are free packages available from NPM for most popular database systems.
If you're just getting started, you might want to stick to MongoDB. It is designed to work well with data in JSON format, which JavaScript is great at working with as well. Also, it is the database system used by most tutorials to teach Node.js, so you'll have access to a greater number of educational materials if you pair your Node.js application with a MongoDB database.
- What is NPM?
NPM, short for Node Package Manager, is a package manager for JavaScript. It includes a repository of JavaScript packages that any developer can incorporate into their projects and gives developers the ability to manage the exact version of each package that is incorporated into each project.
- What are NPM packages?
NPM packages are JavaScript packages designed to accomplish a specific task. For example, there are NPM packages that provide the drivers necessary to connect Node.js to a MySQL database. In effect, packages provide boilerplate code developed by other JavaScript developers that you can incorporate into your projects. That way you can avoid having to program routine tasks and can instead focus on the core logic behind your application.
- What is a runtime environment?
A runtime environment provides an application with all of the resources it needs to operate. For instance, in order to run on a machine, an application will need access to the directories and files on the machine, hardware resources such as RAM and CPU, and software resources such as system utilities and software libraries.
In the context of a webs server, Node.js is a runtime environment that runs a JavaScript application and provides the application with access to all of the server resources necessary to operate.
Comments