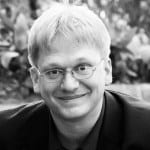
Object-Oriented Programming: Making Complicated Concepts Simple
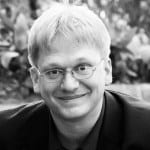
Disclosure: Your support helps keep the site running! We earn a referral fee for some of the services we recommend on this page. Learn more
Object-oriented (OO) programming is a programming paradigm that includes or relies on the concept of objects, encapsulated data structures that have properties and functions and which interact with other objects.
Objects in a program frequently represent real-world objects — for example, an ecommerce website application might have Customer
objects, Shopping_cart
objects, and Product
objects. Other objects might be loosely related to real-world equivalents — like a Payment_processor
or Login_form
. Many other objects serve application logic and have no direct real-world parallel — objects that manage authentication, templating, request handling, or any of the other myriad features needed for a working application.
History of Object-oriented Programming
If it seems like object-oriented programming is particularly well suited to modeling real world systems, that isn’t a coincidence. The concepts now associated with object-oriented programming, including most of the language for describing it, originated with the Simula programming language, which was used for simulating real world systems. Classes, subclasses, and objects were added to Simula in 1967.
In the 1970s, Alan Kay coined the term “object-oriented” while working on Smalltalk. This was in reference to the extensive use of objects in the language, and their place as the fundamental unit of organization.
The spread of object-oriented programming was relatively slow at first. Common Lisp introduced the Common Lisp Object System, adding objects to Lisp, in the late 1970s. In the 1980s, the Eiffel programming language was invented, which was fully object-oriented. The inventor of Eiffel, Bertrand Meyer, wrote Object-Oriented Software Construction, which was one of the first books to widely disseminate the concepts of OO programming.
In the 1990s and into the 2000s, object-oriented programming really took off. This coincided with a massive explosion in the tech industry generally during that period, and the sudden influx of programmers into large tech companies. One of the selling points of object-oriented programming, as promoted by its advocates, is that separation of concerns and loose coupling (see below) enable teams to work on large systems more effectively.
By the end of the first decade of the 21st century, object-oriented programming had largely become the accepted “one right way” of developing most software. Newer programming languages, especially dynamically-typed interpreted languages like Python, Ruby, and JavaScript, tend to assume, or even enforce, an object-oriented approach. Meanwhile, many older languages, like Fortran and COBOL have added object-oriented features.
Important Object-oriented Concepts
Object-oriented programming is a concept — a way of approaching the problem of designing a computer program. It is not a hard-and-fast set of rules or a specific methodology. Different programming languages approach OOP differently. Some are more or less strict, and some are just different. What follows is a general overview of object-oriented design concepts. See our sister article, Object-Oriented Programming for resources and details that explain how these concepts are implemented in many different languages.
Separation of Concerns
The central guiding principle which informs all the other concepts in object-oriented programming is separation of concerns. This is the idea that logically distinct bundles of data and functionality should be separated into distinct bundles of application code.
This idea is not unique to object-oriented programming. Most programming languages allow for separation of concerns, and most programming paradigms promote it. However, separation of concerns is of special importance to object-oriented programming. Strong separation of concerns is the impetus behind nearly all other OO concepts.
Objects and Classes
It seems, at first, that the fundamental concept in object-oriented programming is objects. In a way, that’s true. But from the developer’s perspective, the fundamental concern is classes.
A class defines an object; an object instantiates a class. For an example, let’s keep thinking about an ecommerce website. There are a lot of customers, but they all do the same thing. So there would be a Customer
class, in which the programmer defined what a customer is, and how it works. Then, each individual customer is represented in the system by a customer object.
Since class definitions specify all the implementation details, most of the work of developing an object-oriented program involves working with classes, not objects.
Classical and Non-classical OO Languages
There are a handful of languages which incorporate a large number of object-oriented concepts, but which don’t have a construct specifically called a class. Most notable is JavaScript, which uses prototypes, or generic objects which are then cloned. The terminology, the underlying philosophy, and even the “under the hood” implementation are different — and yet the developer experience is largely the same.
Languages with classes, which represent the majority of OO languages, are sometimes called “classical.” This is both a pun on the word “class,” and an acknowledgement that classes have become the conventional approach to object-oriented programming.
Methods and Properties
An object has variables, which are usually called properties or attributes in most languages. For example, a Customer
object might have properties like shipping_address
or name
.
Objects also have functions, called methods. These are things that the object is capable of doing, and are also defined within the class. For example, the Customer
object might have methods for checkout
, change_shipping_address
, or logout
.
One of the challenges of developing good object-oriented programs is determining how to split up functions among several potential classes. For example, should a checkout
method belong to the Customer
or to the Shopping Cart
? Does the Customer
own the logout
method, or does the Customer
call the logout
method of some Authentication_manager
object?
Another challenge for many new OO programmers is understanding how to design classes that don’t represent real-world things. Understanding that ecommerce needs Products
and a project management system needs Projects
is pretty easy. But large object-oriented programs have countless classes defining all sorts of abstract bundles of functionality, like object relational mappers, object factories, and controllers (just to name three easy-to-understand examples).
Navigating these concerns takes practice, of course. An object-oriented developer can also be aided by an understanding of design patterns, and by a development process that includes modeling.
Here are a few resources to help you better understand class, properties, and methods:
- Classes, Objects, Properties, and Method Concepts is a great 10-minute video overview of these concepts;
- Choosing Between Properties and Methods is a .NET tutorial, covering some design considerations which are applicable to any language;
- Understanding Classes, Methods, and Properties in C# provides a good explanation, with some helpful diagrams.
Message Passing and Dynamic Dispatch
Classes, and a number of other concepts covered here, have become inexorably linked to object-oriented programming. However, according to the computer scientist who coined the term, object-oriented programming is about message passing.
Message passing means that objects communicate with each other by sending messages. This sounds trivial, but it affects how function calls are made. Rather than one object calling another object’s functions directly, an object would pass a message to another object. The message contains a method name and any associated parameters. The called object then has the ability to handle that method call however is needed.
This ability for each object to determine its own response to method calls as needed is called dynamic dispatch. It allows objects to evolve over run time, and respond to messages according to their present state.
Message passing can be a little confusing, especially since most languages in use today only implement one particular kind (the method call). Here are a few resources to help you better understand it:
- Some one asked What is message passing in OO? on StackExchange; some very helpful answers followed;
- Also see What are the key differences between OO in Smalltalk and Java? for some insight into how message passing has changed from the original idea to how it mostly works in contemporary languages;
- Message Passing on the c2 wiki has a detailed, if somewhat chaotic, explanation.
Encapsulation
Closely associated with message passing and dynamic dispatch is the concept of encapsulation. Encapsulation means that code outside of an object cannot directly access its internal data. If, for example, one object needs to “know” a property of another object (for example, a Shipping_manager
might need the shipping_address
of a Customer
object), then the calling object can’t find that data out directly; it has to pass a message “asking” the Customer
object, which then returns the needed data. (This is usually done with a method call.)
Not all object-oriented languages enforce strict encapsulation; some allow an object’s properties to be accessed (get and set) directly. However, many experts in OO development encourage programmers to adhere to encapsulation principles (by not calling properties directly), even in languages that allow it.
There are many benefits to encapsulation. Primarily, it allows any details or side effects to be abstracted away from the point at which the property is called. For example, each time a particular value is read, you might want to check when it was last calculated, and re-calculate it if certain conditions are met. Being able to add, remove, or change that logic in one place at any time, without affecting any of the other places in the code which make the call, is a benefit of encapsulation.
Inheritance and Composition
Inheritance and composition are two ways that various classes (and their instantiated objects) are related to each other.
Inheritance describes “is a” relationships: a User
is a Person
; a Customer
is a User
. In this example, there might be a Person
class which defines all the properties and methods of Persons in general (name
, birthday
). Then a User
class would extend the Person
class, to add things like a username
attribute or login
and logout
methods. A Customer
class might then extend User
, adding those things that only a customer needs, like order_history
or billing_address
.
Composition describes a “has a” relationship, when one object “owns” another object. For example, the Customer
class might own a Billing_address
, which is itself a full-fledged object defined in a class. Like the inheritance hierarchy, the compositional hierarchy can easily be several layers deep. For example: a Customer
might have a Shopping_cart
, the Shopping_cart
has several Products
, each Product
has a Vendor
, and so forth.
Much of the design work in object-oriented development has to do with mapping out these relationships of inheritance and composition. The class diagram, which is a part of the Unified Modeling Language is an invaluable tool for visualizing these relationships.
- Inheritance versus Composition: Which One Should You Choose? goes into some of the design considerations when building a compex class hierarchy;
- Composition vs Inheritance: How to Choose? covers the same question, but from a different angle and with more humor;
- Java Design: Objects, UML, and Process, by Kirk Knoernschild, include a section, “Composite Reuse Principle,” in the first chapter that explains why composition is sometimes better, even when inheritance seems right.
Polymorphism
Polymorphism (or, more specifically, subtype polymorphism) is a concept that naturally comes out of inheritance. If a parent class (or “Super class”) has a particular method, then all of its children (“subclasses”) will also have the method. Each subclass might implement the method differently, but calling objects do not need to know which of several subtypes they are calling. They can treat all different subtypes of objects as equivalent.
Polymorphism is a big subject, and is not without controversy. Here are a few resources to help wrap your head around it:
- Polymorphism in Java — Method Overloading and Overriding is a gentle introduction to polymorphism;
- Object-Oriented Programming Concepts: Polymorphism and Interfaces is specifically about ActionScript, but provides a lot of detail on the subject, including various problems and limitations;
- Polymorphism — Object-oriented Programming in C++ is a nice video explanation;
- The Liskov Substitution Principle (PDF) is a presentation to help you understanding subtyping.
Learn More About Object-oriented Programming
For a deep understanding of object-oriented programming, a number of classic and contemporary books on the subject are invaluable, and can hardly be replaced by online tutorials.
- Design Patterns: Elements of Reusable Object-Oriented Software, by Gamma, et al, is the classic book which introduced object-oriented program to mainstream developers; it is still a must-read;
- Object-Oriented Analysis and Design with Applications, by Booch, et al, goes beyond writing OO code and gets into the meat of the OOP-movement, which is analysis and design of systems;
- The Object-Oriented Thought Process by Matt Weisfeld, is a good book for developers skilled in procedural or functional programming and who need to retrain their thinking toward objects and classes;
- OOP Demystified, by Keogh and Giannini, presents a tutorial on OOP in the context of real world app development, with a focus on the skills and thinking required to apply an object-oriented analysis and design process;
- Object-Oriented Software Construction, by Bertrand Meyer, is a classic book on OO, one of the first to present the technique and philosophy underlying the concepts; some of the material in this book can seem dated, but the fundamentals are still relevant;
- Design Patterns Explained: A New Perspective on Object-oriented Design, by Shalloway and Trott, is the most important book advancing the practice of design patterns, or reusable strategies for tackling recurring problems;
- Object Models: Strategies, Patterns, and Applications, by Coad, North, and Mayfield, is a good companion to Design Patterns Explained, providing many additional patterns and strategies for object-oriented programming.
For a more practical, and contemporary, understanding of object-oriented programming concepts, try one or more of these online resources:
- Quickly Learn Object-oriented Programming is a Python-based tutorial from the Mozilla Developer Network;
- Object-Oriented Programming Concepts is a tutorial from Oracle, covering object-oriented programming basics, from a Java perspective;
- Object-Oriented Programming Tutorial presents a language-agnostic introduction to the core concepts;
- Introduction to Object-Oriented Programming Concepts and More is a great overview of object-oriented programming, and also several closely related subjects, like software architecture and design patterns;
- Review of Object-Oriented Programming from Codecademy, uses JavaScript and an in-browser editor to allow you to immediately work with the concepts (but remember that JavaScript implements OOP concepts a little differently);
- Object-oriented Programming Basics is another Java resource, with a lot of helpful examples with real classes.
Important Object-Oriented Languages
Not all programming languages support object-oriented programming. Some languages are designed to do nothing but support OOP. Others allow for a variety of approaches to programming. Still others appear to be object-oriented, but implement the concepts of object-orientation in non-standard, incomplete, or just plain unusual ways.
Below is a list of some of the more popular OO-capabable languages, with notes about their approach to Object Orientation, and some resources to help you get into OOP with that language.
The internet is filled with essays that look at language X vs language Y — most of which just scratch the surface. We suggest you check out this more general Programming Language Comparison. This thoughtful analysis looks at specific details of several popular OO languages, discussing how particular OO concepts are implemented in each.
C-Based Languages
C is not an object-oriented language. However, it is at least possibleto write OO code in it:
- Object-Oriented Programming with ANSI-C (PDF)
- Phil’s Guide to Object-Oriented ANSI C
- Object-Oriented Programming In C.
None of the techniques described in those books and articles are particularly robust or easy to deal with for non-trivia programs. Rather than trying to stretch C to its limits, there are three direct descendants of C which include object-oriented language tools.
C++
C++ is multi-paradigm, which means that it supports several different programming methods (PDF), including object-orientation. It is based on C, and developed specifically as a way to add support for OOP’s concept of classes.
- Object-Oriented Programming (OOP) in C++: a great intro guide from Computer Science professor
- Learning Object-Oriented Programming in C++: a large, multi-part tutorial on OOP
- C++ Tutorial 20-1 — Classes and Object-Oriented Programming: part of a larger video series on C++, this session begins the coverage on OOP concepts
- Introduction to Object-Oriented Programming Using C++
- Object-Oriented Programming in C++.
C#
C# (“C sharp”) is another C derivative, mostly designed as an improvement over C++ for use in Microsoft’s .NET Framework.
- Object-Oriented Programming in C#
- C# Object-Oriented Programming Basic to Advance (video)
- C# OOPS Concepts (video)
- Object-Oriented Programming Fundamentals in C#: a premium tutorial from PLuralsight (free trial available)
- Introduction to Object-Oriented Programming Concepts in C#: covers abstraction, encapsulation, inheritance and polymorphism in C#
- Top 10 OOPS Concepts In C# .NET With Examples: a great quick guide to orienting yourself in C# if you already understand OOP concepts
- Learning C#: How to Master an Object-Oriented Programming Language: a high-level overview of how to best approach learning C# and OOP in general
- Beginning C# Object-Oriented Programming.
Objective-C
Objective-C was developed about the same time as C++, with essentially the same goal — the addition of OOP capabilities to C. Today, Objective-C is really only used in Apple’s Cocoa Development Platform for OS X and iOS, and GNUstep, its Open Source alternative.
- Object-Oriented Programming with Objective-C
- Intro to Object-Oriented Design
- Object-Oriented Programming and the Objective-C Language (PDF)
- Intro to Object-Oriented Programming.
Common Lisp
Lisp is not essentially Object-Oriented. However Common Lisp, one of the more popular Lisp dialects, includes the Common Lisp Object System (CLOS), which provides OOP features.
The approach to Object-Orientation implemented in CLOS is radically different than the way OO is handled in other languages. This means that CLOS is not a great place to start if you want to learn OO in general, or apply OO in other types of languages. However, if you are getting into Lisp, CLOS is very important. Additionally, if you find OOP especially intriguing, you’ll enjoy studying CLOS to see OO concepts in a different light.
- Fundamentals of CLOS
- A Brief Guide to CLOS
- The Common Lisp Object System: An Overview (PDF)
- CLOS: Integrating Object-Oriented and Functional Programming (PDF)
- Object-Oriented Programming in COMMON LISP: A Programmer’s Guide to CLOS.
Erlang
According to some conventional points of view, Erlang is not an object-oriented language. However, there is a fascinating minority opinion to the contrary, and its proponents state that Erlang Is the Most Object-Oriented Language, or even that Erlang is the only true Object-Oriented language.
Much of this also applies to Elixir, as well. On other hand, maybe this is just OO-style, not true OO.
F#
F# (“F sharp”) is a multi-paradigm language. Its core is really functional programming, but it includes support for OO and attempts to reconcile these two different approaches to programming.
- The “Object-oriented programming in F#” series
- F# Quick Guides: Object-Oriented Programming
- F# Overview — Imperative and Object-Oriented Programming
- Object-Oriented F# — Creating Classes
- Object-oriented Programming in F# (PDF)
- OOP without classes in F#.
Fortran
Fortran is the oldest programming language still in common use. When it was invented in the 1950s, there was no such thing as object orientation. Explicit OOP support was added to Fortran with the Fortran 2003 release of the language. But it was possible to implement some basic OOP concepts in earlier versions of the language.
- OOP in Fortran before Fortran was Object-Oriented:
- OOP in Fortran with official support:
Go
Go is a relatively new language, having been developed by Google in 2007. There is a lot of discussion about whether or not Go is object-oriented.
Go doesn’t have anything in it called “object” or “class,” but it does have some analogous structures. From a certain point of view, then, you can think of Go as object-oriented.
Many people in fact do think that Go is OO:
- Go Object-Oriented Design
- Object-Oriented Programming in Go
- Object-Oriented Language Patters in Go
- Go for Object-Oriented Programmers or Object-Oriented Programming without Objects (video)
- Summary of Object-Oriented Programming Goodness in Go, without the pain.
Java
Java was built from the ground up to be object-oriented. Here are some great resources to get you going with Java:
- Object-Oriented System Design: the website for Stanford’s CS108, with links to code, handouts, lecture slides, and pretty much everything you need to work through the course on your own
- The Java Tutorial: Object-Oriented Programming Concepts
- Java Programming Tutorial Object-oriented Programming (OOP) Basics
- Object-Oriented Programming Concepts (six video series)
- Object-Oriented Programming — Java Tutorial
- Java Fundamentals Tutorial: Object-Oriented Programming in Java
- Object-oriented Programming in Java
- Object-Oriented Programming Basics With Java (PDF).
JavaScript
A lot of people don’t think that JavaScript is really object-oriented. But a lot of other people think that it definitely is.
We feel our job is to help you find useful resources, not take sides in unwinnable debates. So here are some OOP resources for JavaScript:
- Introduction to Object-Oriented JavaScript (Mozilla Developer Network)
- JavaScript: The World’s Most Misunderstood Programming Language
- OOP In JavaScript: What You NEED to Know
- Object-Oriented JavaScript: Create Scalable, Reusable High-Quality JavaScript Applications and Libraries
- The Principles of Object-Oriented JavaScript
- Object-Oriented JavaScript (video).
PHP
PHP did not support even basic OO concepts until version 4, and didn’t support full object-orientation until PHP5.
- Object-Oriented PHP for Beginners
- Object-Oriented PHP for Absolute Beginners
- Principles Of Object-Oriented Programming in PHP
- Object-Oriented PHP (video)
- Object-Oriented PHP: Concepts, Techniques, and Code.
Python
Python codes does not need to be object-oriented, but the language fully supports it. Check out these resources:
- Object-Oriented Programming: an introduction to OOP, by way of Python, from MIT’s Open Courseware
- Object-Oriented Programming in Python
- Improve Your Python: Python Classes and Object-Oriented Programming
- Python Course: Object-Oriented Programming
- Python 3 Object-Oriented Programming.
Ruby
In Ruby everything is an object, even “primitive” data types like strings and integers. You add two integers by calling the addition method on one of them; you find the length of a string by calling the length method on it.
- Ruby User’s Guide: Object-oriented Thinking
- Ruby Module: A Major OOPs Feature of the Ruby Programming Language
- Practical Object-Oriented Design in Ruby.
Smalltalk
Smalltalk is probably the most influential object-oriented language, having had a profound effect on the languages that came later. As a result, it is good to have at least some familiarity with it. These resources will get you started:
- Object-Oriented Programming with Smalltalk (PDF)
- Smalltalk and Object-Orientation: An Introduction (PDF)
- Smalltalk: Getting started with the language (video)
- Programming Smalltalk – Object-Orientation from the Beginning: an Introduction to the Principles of Programming.
On the Other Hand…
If you want to be a well-rounded developer, it is important to understand both the good and bad of any concept. Even though object orientation has become the dominant way of understanding programming, there are legitimate criticisms to be made — both of the ideas themselves, and the culture of hype surrounding them.
Here are a handful of resources presenting the counter-argument to OOP:
- Object-Oriented Programming Is an Expensive Disaster Which Must End
- Object-Oriented Programming Is Inherently Harmful
- Object-Oriented Programming: a Disaster Story.
Object-oriented Programming Today
While object-orientated programming remains an important methodology in computer programming, it no longer holds its place as industry standard for “good software development.” Other paradigms, especially functional programming, are coming to the forefront, along with new languages that support them. Meanwhile criticisms of object-oriented programming, as well as of the design patterns movement, are on the rise.
Still, a large amount of the software written today is object-oriented. If you are working in any of the common object-oriented languages, you need to have a good understanding of basic object-oriented concepts.
More Interesting Stuff
We have more programming guides, tutorials, and infographics related to coding and development:
- C++ Developer Resources: in addition to information about C++, there is more information about object-oriented programming.
- Linux Programming Introduction and Resources: although not specifically about object-oriented programming, this discussion of the many levels of Linux programming is bound to energize you.
- INTERCAL Introduction and Resources: if you find object-oriented programming hard, studying INTERCAL will make it seem easy. This parody (or joke) language is so complicated and horrible that even the deepest discussion of polymorphism will seem pleasant.
What Code Should You Learn?
Confused about what programming language you should learn to code in? Check out our infographic, What Code Should You Learn? It not only discusses different aspects of the languages, it answers important questions such as, “How much money will I make programming Java for a living?”
Text written by Adam Michael Wood. Compiled and edited by Frank Moraes.
Comments